-
Notifications
You must be signed in to change notification settings - Fork 10
DTL.Shape.CellularAutomatonMixIsland (形状クラス)
Gunji Ryota edited this page Jan 15, 2020
·
5 revisions
namespace DTL.Shape {
public class CellularAutomatonMixIsland
}
CellularAutomatonMixIsland
とは "Matrixの描画範囲に1/2の確率で1番目の描画値を設置し、残りの確率で2番目以降の描画値をランダムに設置し、セルオートマトンを指定回数行う" 機能を持つクラスである。
描画範囲の全てを必ず塗りつぶす
なし
Constructors | Descriptions | Version |
---|---|---|
CellularAutomatonMixIsland() | Matrixの内容をそのまま出力するCellularAutomatonMixIslandのインスタンスを生成します。 | v0.1.0 |
CellularAutomatonMixIsland(uint loopNum, IList list) | 描画値とセルオートマトンの回数を指定したCellularAutomatonMixIslandのインターフェースを生成します。 | v0.1.0 |
CellularAutomatonMixIsland(DTL.Base.Coordinate2DimensionalAndLength2DimensionalmatrixRange, uint loopNum, IList list) | 描画範囲と描画値, セルオートマトンの回数を指定したCellularAutomatonMixIslandのインスタンスを生成します。 | v0.1.0 |
なし
Name | Descriptions | Version |
---|---|---|
GetPointX | 描画始点座標Xを取得 | v0.1.0 |
GetPointY | 描画始点座標Yを取得 | v0.1.0 |
GetWidth | 描画横幅Wを取得 | v0.1.0 |
GetHeight | 描画縦幅Hを取得 | v0.1.0 |
Name | Descriptions | Version |
---|---|---|
SetPointX | 描画始点座標Xを指定 | v0.1.0 |
SetPointY | 描画始点座標Yを指定 | v0.1.0 |
SetWidth | 描画横幅Wを指定 | v0.1.0 |
SetHeight | 描画縦幅Hを指定 | v0.1.0 |
SetPoint | 描画始点座標(X,Y)を指定 | v0.1.0 |
SetRange | 描画範囲(X,Y,W,H)を指定 | v0.1.0 |
名前 | 説明 | 対応バージョン |
---|---|---|
ClearPointX | 描画始点座標Xを消去 | v0.1.0 |
ClearPointY | 描画始点座標Yを消去 | v0.1.0 |
ClearWidth | 描画横幅Wを消去 | v0.1.0 |
ClearHeight | 描画縦幅Hを消去 | v0.1.0 |
ClearPoint | 描画始点座標(X,Y)を消去 | v0.1.0 |
ClearRange | 描画範囲(X,Y,W,H)を消去 | v0.1.0 |
ClearValue | 描画値を消去 | v0.1.0 |
Name | Descriptions | Version |
---|---|---|
Draw(int[,]) | Matrixに描画する | v0.1.0 |
Create(int[,]) | Matrixに描画してMatrixを返す | v0.1.0 |
using System.Collections.Generic;
using UnityEngine;
using DTL.Shape;
using DTL.Console;
public class CellularAutomatonMixIslandGenerator : MonoBehaviour {
public int width = 50;
public int height = 50;
public uint loopNum = 5;
public List<int> list = new List<int>();
CellularAutomatonMixIsland cellularAutomatonMixIsland;
void Start () {
var matrix = new int[height, width];
cellularAutomatonMixIsland = new CellularAutomatonMixIsland(loopNum, list);
cellularAutomatonMixIsland.Draw(matrix);
new OutputConsole().Draw(matrix);
}
}
0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0
0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 4 0 0 0 0 3 0 3 3 3 0
0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 4 4 0 0 0 0 3 3 3 3 0 0
0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 3 3 3 3 3 0
0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 3 0 0 5 0 0 0 0 0 1 0 0 0 3 0
0 0 0 4 0 0 0 0 0 0 0 0 0 0 0 4 3 3 5 0 0 0 0 1 0 0 1 0 0 0 3 0
0 4 4 4 4 4 0 0 0 0 0 0 0 0 2 2 3 3 5 0 0 0 0 1 1 1 1 0 0 0 0 0
0 4 4 4 4 0 0 0 0 0 0 0 4 2 2 0 0 0 0 0 0 0 0 1 1 1 0 0 0 0 0 0
0 0 0 4 4 0 0 0 0 0 0 0 4 2 2 0 3 2 3 0 0 0 1 1 1 1 1 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0 0 0 2 0 0 0 2 0 3 3 0 1 1 1 0 1 0 0 0 0 0
0 0 0 0 4 0 4 0 0 1 0 0 1 2 0 2 0 2 0 1 1 0 1 0 1 0 1 1 1 1 0 0
0 0 5 4 4 4 4 4 1 1 0 0 0 2 0 0 0 0 0 1 1 0 1 0 0 0 0 1 1 0 0 0
0 0 0 5 5 0 0 0 3 3 0 0 3 0 0 0 0 0 0 0 1 0 0 0 0 3 3 3 0 0 0 0
0 0 0 0 4 0 0 0 0 3 3 3 3 0 0 0 3 3 3 0 0 0 0 0 0 0 3 0 0 0 0 0
0 0 0 4 0 1 0 3 0 3 3 3 3 0 0 3 3 3 3 3 0 0 0 0 0 2 2 2 0 0 0 0
0 0 0 0 0 1 0 3 3 3 1 3 3 3 3 3 3 3 3 3 0 0 0 0 0 2 2 2 2 0 0 0
0 4 4 4 4 3 3 3 3 3 3 3 0 3 3 3 3 3 0 0 0 0 0 0 0 0 0 0 2 0 0 0
0 4 0 3 4 1 1 3 3 3 1 0 0 0 3 5 3 3 0 4 0 0 0 0 0 0 0 0 0 0 0 0
0 4 3 4 3 3 1 1 1 1 0 0 0 3 3 5 5 5 0 0 0 0 0 0 4 3 0 0 0 0 0 0
0 0 0 0 3 3 3 1 3 0 0 0 0 3 3 5 5 5 5 5 0 0 0 0 0 0 0 0 0 0 0 0
0 4 0 4 3 0 0 1 0 0 0 0 0 3 5 0 0 5 5 0 0 0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 5 5 0 0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0
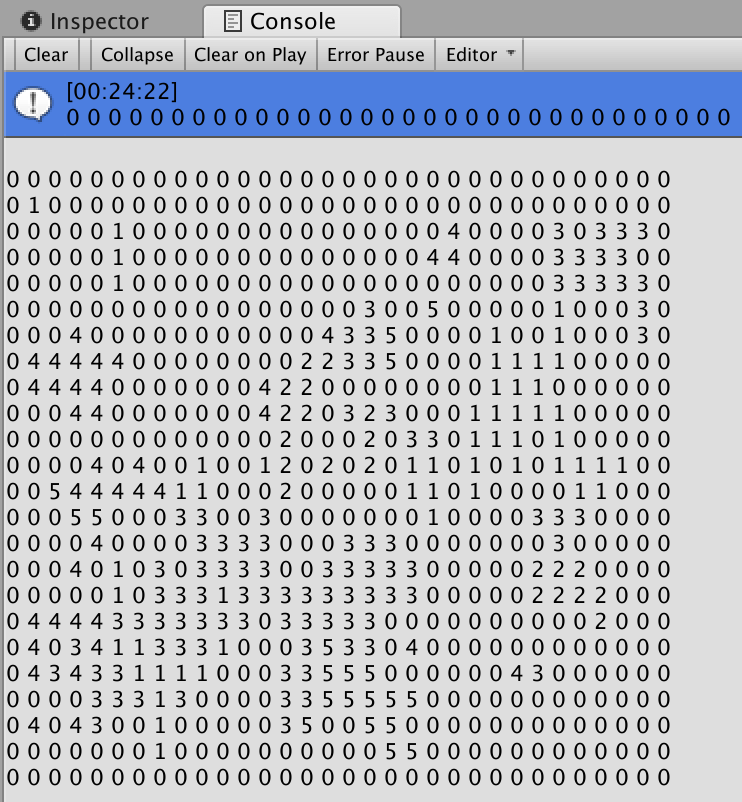
Copyright (c) 2017-2019 Kasugaccho. Copyright (c) 2018-2019 As Project.
Distributed under the Boost Software License, Version 1.0.(See accompanying file LICENSE_1_0.txt or copy at http://www.boost.org/LICENSE_1_0.txt)