-
Notifications
You must be signed in to change notification settings - Fork 28.9k
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
debug/variables: autofill input from an LRU (#224531)
* debug: improve behavior for slow-running prelaunch tasks Shows this notification if a prelaunch task has been running for more than 10 seconds: 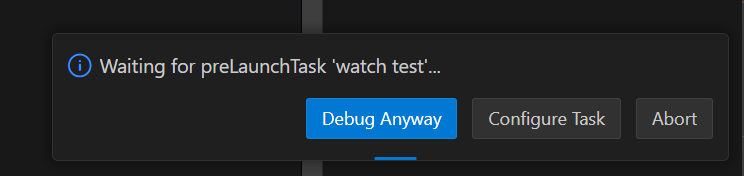 Also does a little cleanup of some probably-leaking disposables in the task runner. Fixes #218267 * hygenie * debug/variables: autofill input from an LRU This keeps a small LRU in the config resolver and storage service to provide default values, when not otherwise specified, for inputs in e.g. debugging. I added this after I was annoyed by having to keep autofilling the same ephemeral value in some debug configs earlier.
- Loading branch information
1 parent
a3bdeb3
commit 930be4a
Showing
4 changed files
with
55 additions
and
16 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters