-
Notifications
You must be signed in to change notification settings - Fork 525
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Merge the preprocess step into the easydeploy onnx/tensorrt model #742
base: dev
Are you sure you want to change the base?
Conversation
TensorRT does not support uint8 input. |
Thanks to point out that issue. I'll fix the uint8 input to float32.
The deployment should FIX not only the structure, but also all the WEIGH/PARAMETER into one file. However in previous mmyolo deployment, we have to read the preprocess WEIGHT again from the config file when doing inferece. It's not a good way, nor friendly for deployment. The deployed model should be decoupled with the config file as much as possible. |
I saw the previous the input normalization was embedded in the |
Any more comment? |
Thanks for your contribution and we appreciate it a lot. The following instructions would make your pull request more healthy and more easily get feedback. If you do not understand some items, don't worry, just make the pull request and seek help from maintainers.
Motivation
Embed the preprocess operation (image_rgb - mean / std ) into the onnx/tensorrt model. So that 1. no need to repeat this preprocess in many inference tasks. 2. The image_rgb can directly fit into the onnx/tensorrt model.
将 RGB normalization 的这一步操作也合并到onnx模型中,1. 在下游图片、视频处理任务中不用再重复preprocess 操作。导出后也不再依赖 config 文件里的mean、std参数。可以减少数据预处理的计算负载。2. 可以直接将 image rgb 的 uint8 数据 (NCHW) 扔给ONNX模型,更方便部署。不影响精度。
Modification
Export the
class PreProcess(torch.nn.Module)
into the deploying model.Main change.
Fit the image_rgb data into the model
The test code below. It didn't change the API at all.
Inspect the ONNX model. You see the model allow rgb uint8 inputs.
Inspect the onnx model.
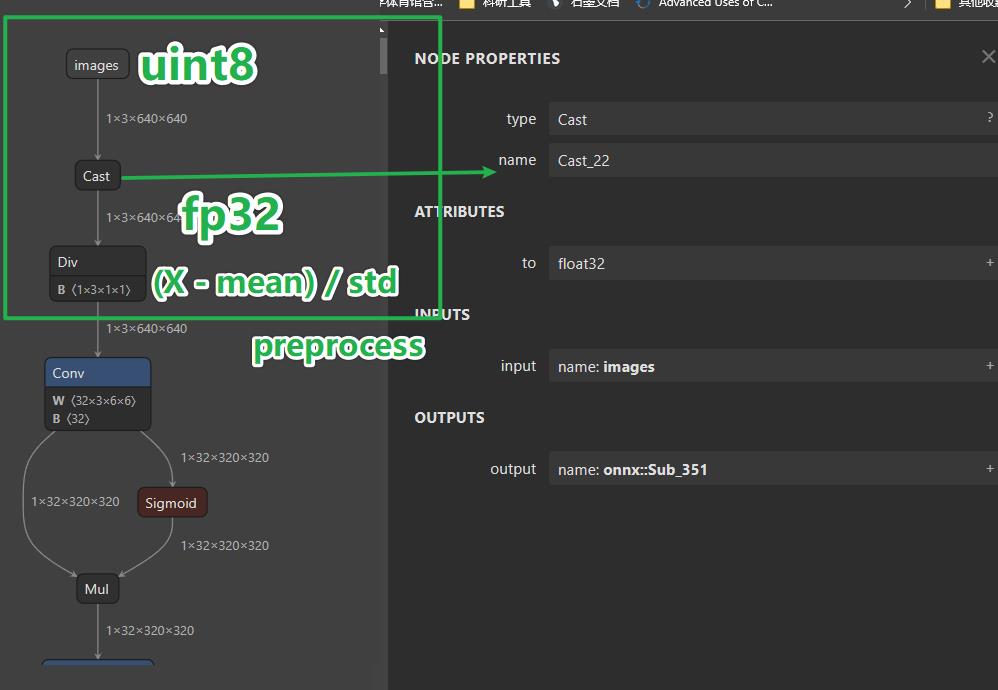
The result compare with original version.
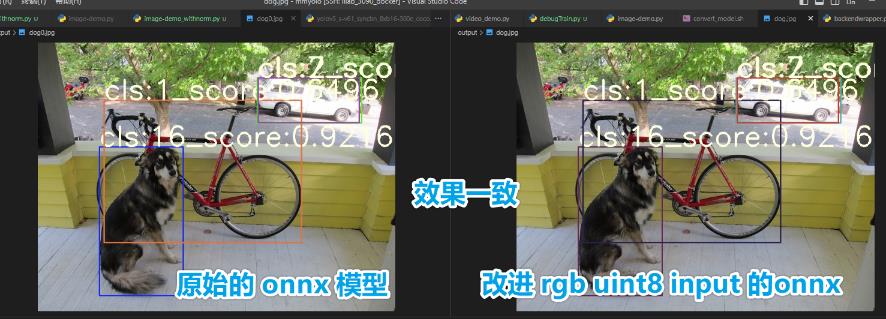
Checklist