-
Notifications
You must be signed in to change notification settings - Fork 3
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
27 changed files
with
1,055 additions
and
99 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,10 +1,19 @@ | ||
Interactive Math Pad | ||
==================== | ||
|
||
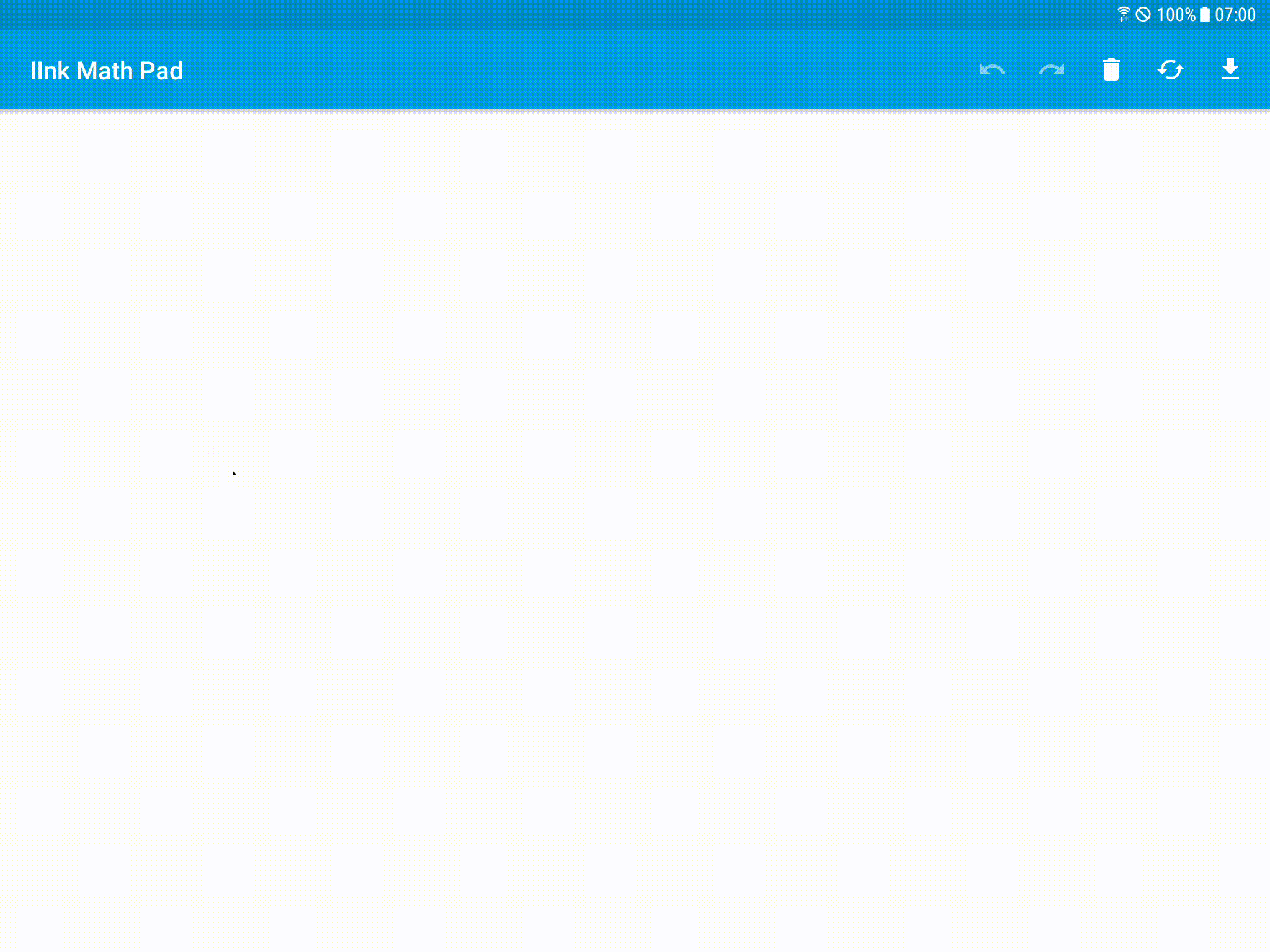 | ||
This is a reproduction of [MyScript MathPad](https://www.myscript.com/retired-apps) on Android platform, based on [MyScript Interactive Ink SDK](https://www.myscript.com/interactive-ink). | ||
|
||
> [!INFO] | ||
> This is a reproduction of the retired MyScript MathPad for Android. | ||
 | ||
|
||
> [!ACKNOWLEDGEMENTS] | ||
> | ||
> - [1] [MyScript Interactive Ink](https://www.myscript.com/interactive-ink)® | ||
> - [2] [中國色](http://zhongguose.com/) | ||
> | ||
> [!DISCLAIMER] | ||
> The software is for your study and reference only and the developer will not be responsible for its content. | ||
> **The software is for your study and reference only and the developer will not be responsible for its content.** | ||
LICENSE | ||
------- | ||
|
||
[MIT License](LICENSE). |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,33 @@ | ||
/* | ||
* Copyright (c) MyScript. All rights reserved. | ||
*/ | ||
|
||
package com.myscript.iink.extensions | ||
|
||
import android.content.ClipData | ||
import android.content.ClipboardManager | ||
import android.content.Context | ||
import android.support.annotation.NonNull | ||
import android.widget.Toast | ||
import com.myscript.iink.Editor | ||
import com.myscript.iink.MimeType | ||
import kotlinx.coroutines.Dispatchers | ||
import kotlinx.coroutines.GlobalScope | ||
import kotlinx.coroutines.launch | ||
|
||
fun Editor.convert() = | ||
getSupportedTargetConversionStates(null).firstOrNull()?.let { convert(null, it) } | ||
|
||
fun Editor.copyToClipboard(@NonNull context: Context, type: MimeType) = | ||
export_(null, type).let { | ||
GlobalScope.launch(Dispatchers.Main) { | ||
(context.getSystemService(Context.CLIPBOARD_SERVICE) as? ClipboardManager)?.run { | ||
primaryClip = ClipData.newPlainText(type.name, it) | ||
Toast.makeText( | ||
context, | ||
"String ($type) copied to clipboard:\n$it", | ||
Toast.LENGTH_LONG | ||
).show() | ||
} | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,14 @@ | ||
<!-- | ||
~ Copyright (c) MyScript. All rights reserved. | ||
--> | ||
|
||
<vector xmlns:android="http://schemas.android.com/apk/res/android" | ||
android:width="24dp" | ||
android:height="24dp" | ||
android:tint="?android:attr/colorControlNormal" | ||
android:viewportWidth="24.0" | ||
android:viewportHeight="24.0"> | ||
<path | ||
android:fillColor="#FF000000" | ||
android:pathData="M6,19c0,1.1 0.9,2 2,2h8c1.1,0 2,-0.9 2,-2V7H6v12zM19,4h-3.5l-1,-1h-5l-1,1H5v2h14V4z" /> | ||
</vector> |
Oops, something went wrong.