-
Notifications
You must be signed in to change notification settings - Fork 4.7k
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Inliner: Extend IL limit for profiled call-sites, allow inlining for switches. #55478
Merged
Merged
Changes from 15 commits
Commits
Show all changes
21 commits
Select commit
Hold shift + click to select a range
d711b0f
Improve Inliner, vol.3
EgorBo 42dcb3d
Merge branch 'main' of https://github.com/dotnet/runtime into jit-inl…
EgorBo 154bd99
Improvements
EgorBo 6dd92ca
Tuning.
EgorBo 4cb8387
Fix asserts
EgorBo 8117d8f
Clean up
EgorBo 5a3effa
Fix assert
EgorBo 36f91ad
Merge branch 'main' of https://github.com/dotnet/runtime into jit-inl…
EgorBo 40588ff
Tuning.
EgorBo e19f79f
add missing break;
EgorBo 2240f34
Tuning for size.
EgorBo d8f4c45
Tuning.
EgorBo f6e7c13
Formatting
EgorBo 970a2cd
Tuning.
EgorBo a7ecdf6
Revert some changes
EgorBo db0f3bc
Fix cases where "arg op cns" left "cns" on top of the pushedstack -- …
EgorBo d2bbe6d
Merge branch 'jit-inliner-3' of https://github.com/EgorBo/runtime-1 i…
EgorBo a382443
Merge branch 'main' of https://github.com/dotnet/runtime into jit-inl…
EgorBo 4d0bf98
Fix an assert when we import a switch (in jitdump mode)
EgorBo e948d10
Disable over-ILsize for generic static pgo
EgorBo ab9f542
Update fgbasic.cpp
EgorBo File filter
Filter by extension
Conversations
Failed to load comments.
Loading
Jump to
Jump to file
Failed to load files.
Loading
Diff view
Diff view
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.
Add this suggestion to a batch that can be applied as a single commit.
This suggestion is invalid because no changes were made to the code.
Suggestions cannot be applied while the pull request is closed.
Suggestions cannot be applied while viewing a subset of changes.
Only one suggestion per line can be applied in a batch.
Add this suggestion to a batch that can be applied as a single commit.
Applying suggestions on deleted lines is not supported.
You must change the existing code in this line in order to create a valid suggestion.
Outdated suggestions cannot be applied.
This suggestion has been applied or marked resolved.
Suggestions cannot be applied from pending reviews.
Suggestions cannot be applied on multi-line comments.
Suggestions cannot be applied while the pull request is queued to merge.
Suggestion cannot be applied right now. Please check back later.
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
This used to be 1000 -- I assume you're increasing this to keep prejit image size small?
If so, you should add a comment describing how this value influences prejit size.
If not, you might comment on what the impact of changing this would be.
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
1000 samples feels like a plenty of evidence that something is hot.
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Reverted to 1000. Yes, this changes was supposed to decrease the prejitted size, I was using this histogram:
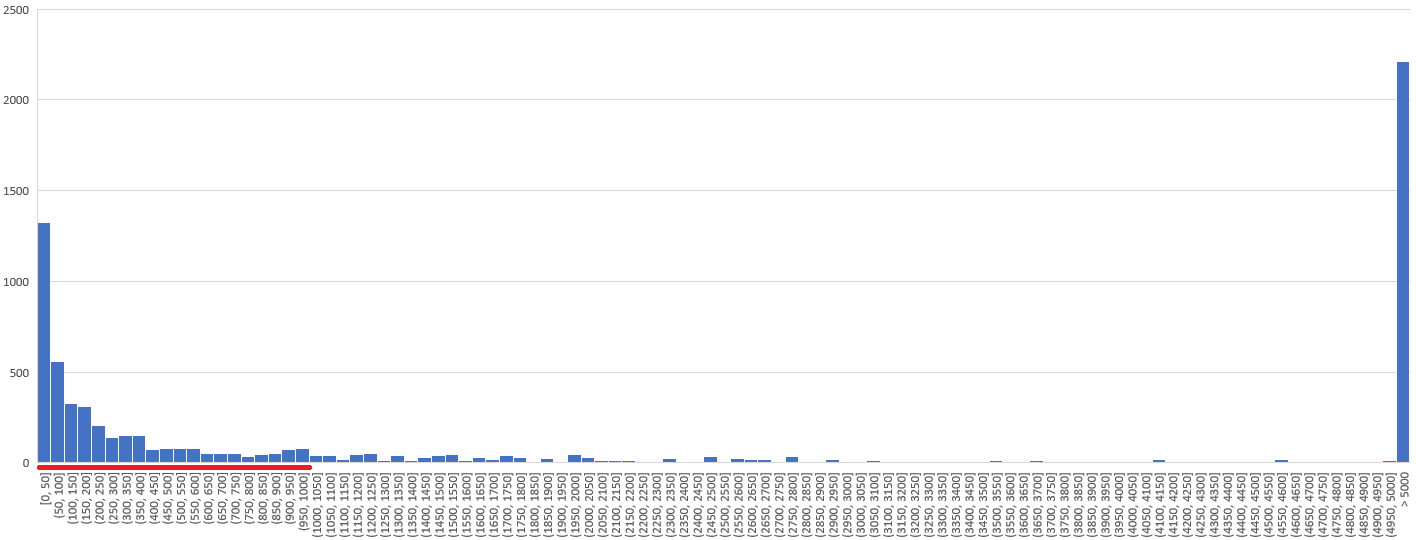
(weights in SPC, the right column starts at 50000).
However, I don't need to save some space with it anymore as I've found an unrelated issue that bloated size for no reason (binary expressions like "arg op cns" used to leave "cns" on top of the pushed stack so there were lots of false-positive foldable-branches/switches).