diff --git a/README.md b/README.md
index 0e7379f3..d6cbb6bb 100644
--- a/README.md
+++ b/README.md
@@ -2,14 +2,18 @@
[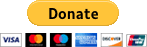](https://www.paypal.me/iqoptionapi)
-last Version:3.6.4
+last Version:3.7
-last update:2019/4/27
+last update:2019/8/13
-version 3.6.4
-
-[add multi buy function](#buymulti)
+[buy current price](#buydigitalspot)
+[change strike_list api](#strikelist)
+need duration time
+```python
+subscribe_strike_list(ACTIVES,duration)
+unsubscribe_strike_list(ACTIVES,duration)
+```
---
## About API
@@ -387,7 +391,7 @@ I_want_money=IQ_Option("email","password")
ACTIVES="EURUSD"
duration=1#minute 1 or 5
amount=1
-I_want_money.subscribe_strike_list(ACTIVES)
+I_want_money.subscribe_strike_list(ACTIVES,duration)
#get strike_list
data=I_want_money.get_realtime_strike_list(ACTIVES, duration)
print("get strike data")
@@ -429,11 +433,11 @@ if buy_check:
else:
print("you loose")
break
- I_want_money.unsubscribe_strike_list(ACTIVES)
+ I_want_money.unsubscribe_strike_list(ACTIVES,duration)
else:
print("fail to buy,please run again")
```
-#### Get all strike list data
+#### Get all strike list data
##### Data format
@@ -450,13 +454,29 @@ import time
I_want_money=IQ_Option("email","password")
ACTIVES="EURUSD"
duration=1#minute 1 or 5
-I_want_money.subscribe_strike_list(ACTIVES)
+I_want_money.subscribe_strike_list(ACTIVES,duration)
while True:
data=I_want_money.get_realtime_strike_list(ACTIVES, duration)
for price in data:
print("price",price,data[price])
time.sleep(5)
-I_want_money.unsubscribe_strike_list(ACTIVES)
+I_want_money.unsubscribe_strike_list(ACTIVES,duration)
+```
+
+#### buy_digital_spot
+
+buy the digit in current price
+
+```python
+from iqoptionapi.stable_api import IQ_Option
+
+I_want_money=IQ_Option("email","password")
+
+ACTIVES="EURUSD"
+duration=1#minute 1 or 5
+amount=1
+action="call"#put
+print(I_want_money.buy_digital_spot(ACTIVES,amount,action,duration))
```
#### Buy digit
diff --git a/iqoptionapi/api.py b/iqoptionapi/api.py
index e29657d8..1cfd2e5e 100644
--- a/iqoptionapi/api.py
+++ b/iqoptionapi/api.py
@@ -50,7 +50,7 @@
from iqoptionapi.ws.chanels.subscribe import Subscribe_Instrument_Quites_Generated
from iqoptionapi.ws.chanels.unsubscribe import Unsubscribe_Instrument_Quites_Generated
-
+from iqoptionapi.ws.chanels.digital_option import Digital_options_place_digital_option
from iqoptionapi.ws.chanels.api_game_getoptions import Getoptions
from iqoptionapi.ws.chanels.sell_option import Sell_Option
from iqoptionapi.ws.chanels.change_tpsl import Change_Tpsl
@@ -110,6 +110,8 @@ class IQOptionAPI(object): # pylint: disable=too-many-instance-attributes
close_position_data=None
overnight_fee=None
#---for real time
+ digital_option_placed_id=None
+
real_time_candles=nested_dict(3,dict)
real_time_candles_maxdict_table=nested_dict(2,dict)
candle_generated_check=nested_dict(2,dict)
@@ -445,7 +447,9 @@ def subscribe_instrument_quites_generated(self):
def unsubscribe_instrument_quites_generated(self):
return Unsubscribe_Instrument_Quites_Generated(self)
-
+ @property
+ def place_digital_option(self):
+ return Digital_options_place_digital_option(self)
#____BUY_for__Forex__&&__stock(cfd)__&&__ctrpto_____
@property
diff --git a/iqoptionapi/stable_api.py b/iqoptionapi/stable_api.py
index eefbd562..3e6706e6 100644
--- a/iqoptionapi/stable_api.py
+++ b/iqoptionapi/stable_api.py
@@ -5,6 +5,8 @@
import time
import logging
import operator
+import datetime
+import pytz
from collections import defaultdict
@@ -16,7 +18,7 @@ def nested_dict(n, type):
class IQ_Option:
- __version__ = "3.6.4"
+ __version__ = "3.7"
def __init__(self, email, password):
self.size = [1, 5, 10, 15, 30, 60, 120, 300, 600, 900, 1800,
@@ -632,12 +634,12 @@ def get_strike_list(self, ACTIVES, duration):
return self.api.strike_list, None
return self.api.strike_list, ans
- def subscribe_strike_list(self, ACTIVE):
- self.api.subscribe_instrument_quites_generated(ACTIVE)
+ def subscribe_strike_list(self, ACTIVE,expiration_period):
+ self.api.subscribe_instrument_quites_generated(ACTIVE,expiration_period)
- def unsubscribe_strike_list(self, ACTIVE):
+ def unsubscribe_strike_list(self, ACTIVE,expiration_period):
del self.api.instrument_quites_generated_data[ACTIVE]
- self.api.unsubscribe_instrument_quites_generated(ACTIVE)
+ self.api.unsubscribe_instrument_quites_generated(ACTIVE,expiration_period)
def get_realtime_strike_list(self, ACTIVE, duration):
while True:
@@ -674,6 +676,32 @@ def get_realtime_strike_list(self, ACTIVE, duration):
pass
return ans
+ #thank thiagottjv
+ #https://github.com/Lu-Yi-Hsun/iqoptionapi/issues/65#issuecomment-513998357
+ def buy_digital_spot(self, active,amount, action, duration):
+ #Expiration time need to be formatted like this: YYYYMMDDHHII
+ #And need to be on GMT time
+
+ UTC=datetime.datetime.utcnow()
+ dateFormated = str(UTC.strftime("%Y%m%d%H"))+str(int(UTC.strftime("%M"))+duration )
+
+ #Type - P or C
+ if action == 'put':
+ action = 'P'
+ elif action=='call':
+ action = 'C'
+ else:
+ logging.error('buy_digital_spot active error')
+ return -1
+ #doEURUSD201907191250PT5MPSPT
+ instrument_id = "do" + active + dateFormated + "PT" + str(duration) + "M" + action + "SPT"
+ self.api.digital_option_placed_id=None
+
+ self.api.place_digital_option(instrument_id,amount)
+ while self.api.digital_option_placed_id==None:
+ pass
+
+ return self.api.digital_option_placed_id
def buy_digital(self, amount, instrument_id):
self.api.position_changed = None
diff --git a/iqoptionapi/ws/chanels/digital_option.py b/iqoptionapi/ws/chanels/digital_option.py
new file mode 100644
index 00000000..04ada9a3
--- /dev/null
+++ b/iqoptionapi/ws/chanels/digital_option.py
@@ -0,0 +1,23 @@
+#python
+
+import datetime
+import time
+from iqoptionapi.ws.chanels.base import Base
+
+#work for forex digit cfd(stock)
+
+class Digital_options_place_digital_option(Base):
+ name = "sendMessage"
+ def __call__(self,instrument_id,amount):
+ data = {
+ "name": "digital-options.place-digital-option",
+ "version":"1.0",
+ "body":{
+ "user_balance_id":int(self.api.profile.balance_id),
+ "instrument_id":str(instrument_id),
+ "amount":str(amount)
+
+ }
+ }
+ self.send_websocket_request(self.name, data)
+
diff --git a/iqoptionapi/ws/chanels/subscribe.py b/iqoptionapi/ws/chanels/subscribe.py
index e358d408..0b8a6fed 100644
--- a/iqoptionapi/ws/chanels/subscribe.py
+++ b/iqoptionapi/ws/chanels/subscribe.py
@@ -44,12 +44,13 @@ def __call__(self, active_id):
class Subscribe_Instrument_Quites_Generated(Base):
name = "subscribeMessage"
- def __call__(self,ACTIVE):
+ def __call__(self,ACTIVE,expiration_period):
data = {
"name": "instrument-quotes-generated",
"params":{
"routingFilters":{
"active":int(OP_code.ACTIVES[ACTIVE]),
+ "expiration_period":int(expiration_period*60),
"kind":"digital-option",
},
diff --git a/iqoptionapi/ws/chanels/unsubscribe.py b/iqoptionapi/ws/chanels/unsubscribe.py
index 6e50ac99..171acef4 100644
--- a/iqoptionapi/ws/chanels/unsubscribe.py
+++ b/iqoptionapi/ws/chanels/unsubscribe.py
@@ -43,12 +43,13 @@ def __call__(self, active_id,size=1):
class Unsubscribe_Instrument_Quites_Generated(Base):
name = "unsubscribeMessage"
- def __call__(self,ACTIVE):
+ def __call__(self,ACTIVE,expiration_period):
data = {
"name": "instrument-quotes-generated",
"params":{
"routingFilters":{
"active":int(OP_code.ACTIVES[ACTIVE]),
+ "expiration_period":int(expiration_period*60),
"kind":"digital-option",
},
},
diff --git a/iqoptionapi/ws/client.py b/iqoptionapi/ws/client.py
index 5e133c85..e8bc89d0 100644
--- a/iqoptionapi/ws/client.py
+++ b/iqoptionapi/ws/client.py
@@ -188,6 +188,12 @@ def on_message(self, wss, message): # pylint: disable=unused-argument
self.api.position_changed=message
elif message["name"]=="auto-margin-call-changed":
self.api.auto_margin_call_changed_respond=message
+ elif message["name"]=="digital-option-placed":
+ try:
+ self.api.digital_option_placed_id=message["msg"]["id"]
+ except:
+ self.api.digital_option_placed_id="error"
+
elif message["name"]=="instrument-quotes-generated":
Active_name=list(OP_code.ACTIVES.keys())[list(OP_code.ACTIVES.values()).index(message["msg"]["active"])]
period=message["msg"]["expiration"]["period"]
diff --git a/setup.py b/setup.py
index 8c50684a..d6d0b7e4 100644
--- a/setup.py
+++ b/setup.py
@@ -3,7 +3,7 @@
setup(
name="iqoptionapi",
- version="3.6.4",
+ version="3.7",
packages=find_packages(),
install_requires=["pylint","requests","websocket-client==0.47"],
include_package_data = True,